Using the Bluemix Insights for Twitter Service with a Rails App
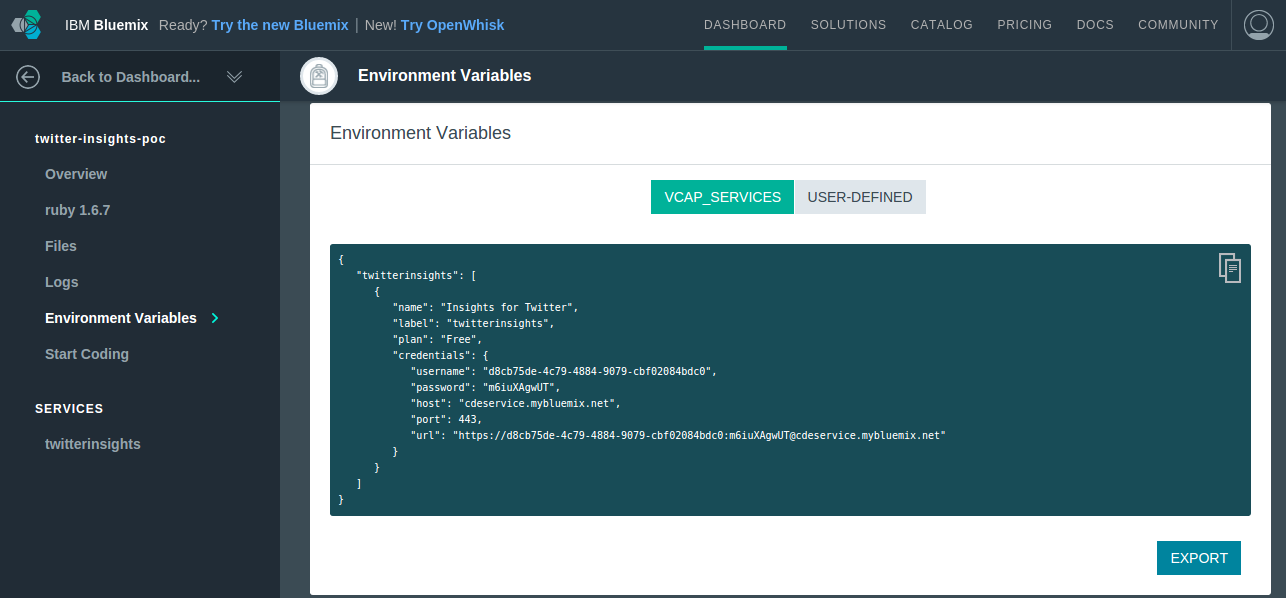
The tutorial also provides sample code for a proof of concept.
A step-by-step tutorial
Today, I will show how to use one of the many services provided by Bluemix in a Rails application.
It is a really easy process once you get the idea. Here is my working code at GitHub. Now, let’s go through the main steps.
- Create a Ruby web application from the Bluemix dashboard.
- Create the Insights for Twitter service and bind it to your application.
- Click your application on the Bluemix dashboard, go to the Start Coding section, and follow the instructions for downloading your application.
- Run
bundle install
. Install the Figaro gem following these instructions and add the necessary environment variables to the
config/application.yml
file.To find these variables, go to your application summary on the Bluemix dashboard and click Show Credentials on the Insights for Twitter service tile. Once there, the needed variables are the ones inside the
credentials
key. All environment information related to services bound to your application is stored in theVCAP_SERVICES
environment variable.Here are these values in your Bluemix application console.
I added the variables required for connecting to the Insights for Twitter service, and here is my sample file application.yml.sample. The variables from this file will be automatically set as environment variables. The file should be ignored by Git, but it will be uploaded to your Bluemix instance unless you create a
.cfignore
file with the files and folders you don’t want to be uploaded.If you don’t want to use the file, you can configure the same environment variables inside the Bluemix user interface. For doing so, click your application tile, go to Environment Variables on the left navigation pane, and click USER-DEFINED.
You will be able to access these variables with
Figaro.env.key_name
or withENV["key_name"]
.Create a class for communicating with the Twitter service.
I decided to create it inside the
services
folder here. Remember to add theservices
folder toapplication.rb
for autoloading it:1config.autoload_paths << Rails.root.join('app/services')Here is the code for the controller:
searches_controller.rb
.For the front end, use the example provided in the service documentation and available at https://cdetestapp.mybluemix.net/.
You can download the
.html
,.css
,.js
, and image files with your browser or from the code I provide.Then, create a new controller (for example,
SearchesController
), create an action inside it (for example,query
), its view (for example,query.html.erb
), and fill it in with the downloaded HTML.You can copy the images,
.css
, and.js
files to theassets
folder and include them properly in the view.Make that action the root of your application by changing
routes.rb
:1root :to => 'search#query'Add another endpoint to
SearchesController
calledcount_tweets
and the corresponding route.This endpoint will use our class service and return a JSON response. Using a class service is the suggested way of making requests. If you make them directly from the browser as in the example given, you will probably have problems with CORS, and you will be exposing sensitive information.
Finally, you will need to change the URL used in the
countTweets()
function inside the JavaScript file to work with our previously created endpoint (count_tweets
).You won’t be able to use the URL helpers directly inside the JavaScript file, so you can define a JavaScript variable with that URL in your
html.erb
and then use it in the .js file.Here are the HTML and JS views:
Also, you could use the gem from https://github.com/gazay/gon for solving the problem of sending Rails variables to JS files or whatever you want.
- Run
cf push app-name
.
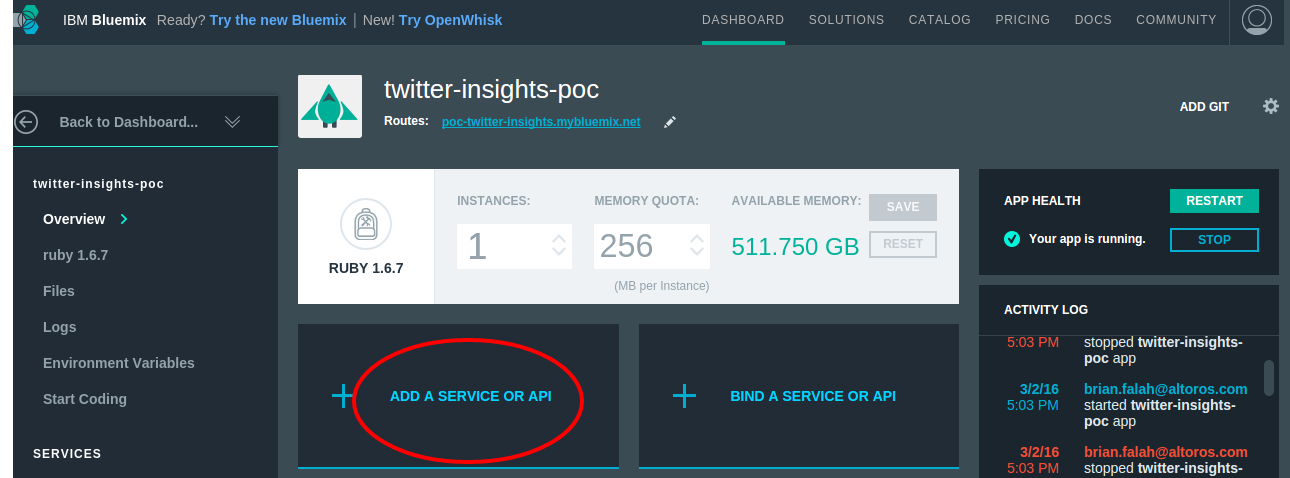
It should work!
Conclusion
I think there’s no big difference between using a service provided by Bluemix and services from other vendors when what you are doing is a simple call to an API.
As for me, the most important advantages of such services are the easiness of subscription, the fact that they provide you with credentials in an organized and standardized way, and a possible discount because of buying them from Bluemix.
Related reading
- Getting Started with IBM Bluemix: Deploying a Sample Ruby/Sinatra App
- Continuous Integration and Continuous Delivery in IBM Bluemix
- Using IBM Bluemix Object Storage in Ruby Projects
- Deploying a Rails App with Elasticsearch to IBM Bluemix
- Building Java Apps with IBM Bluemix and DB2
- How to Connect to XPages NoSQL DB from the Node.js Runtime in Bluemix