Things that I Hate as a Web Developer
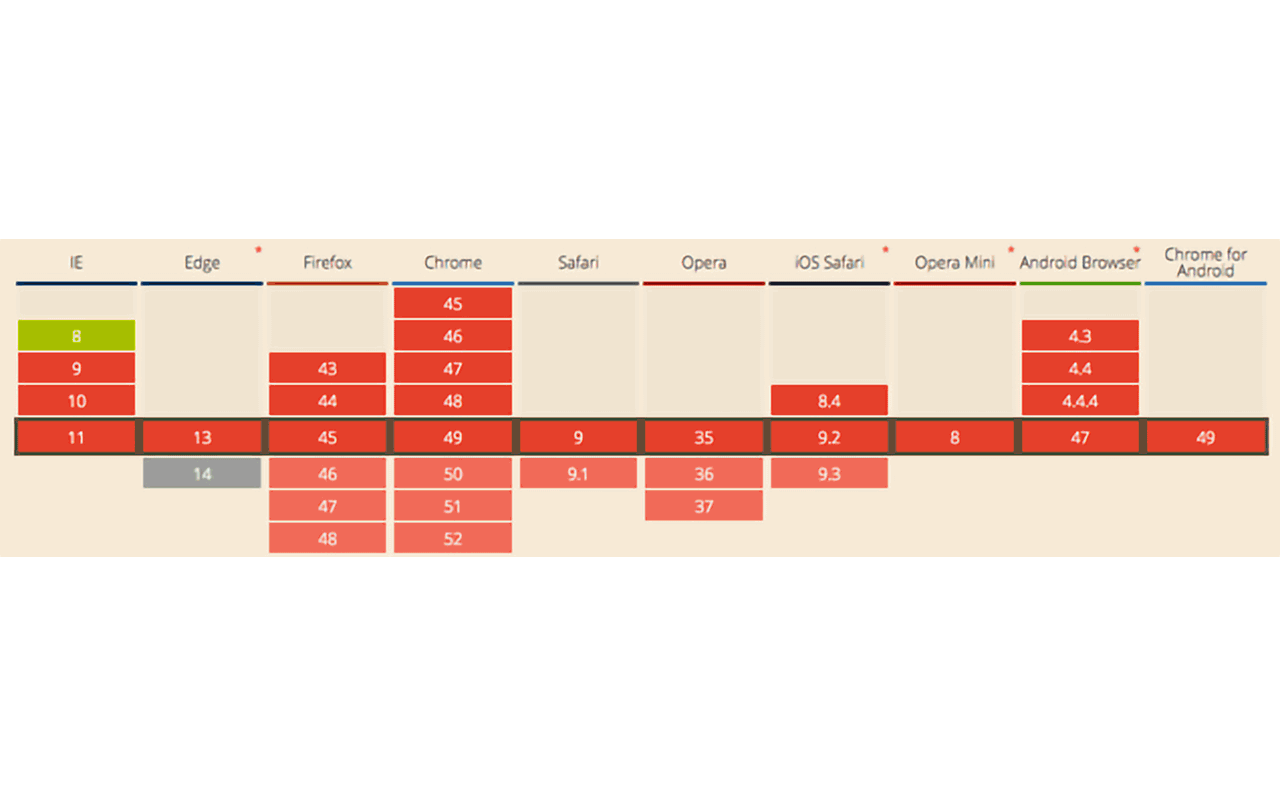
Do you remember your feelings when you had to customize activeadmin
, faced a binding problem in Angular, or read about primitives
for the first time? There are a lot of things that irritate us as web developers. Here is my list. Feel free to add your items in the comments.
Top challenges
Do we really need deep
functions?
Ruby, Ruby on Rails, Lodash, etc., have a lot of similar functions that work in the same way, but with full depth. I can’t imagine a situation when I need to copy just the first level of a hash or an array and continue by using deep
tails in links. Anyway, if there are such cases, a name for a method should be merge_first_level
instead of just merge
.
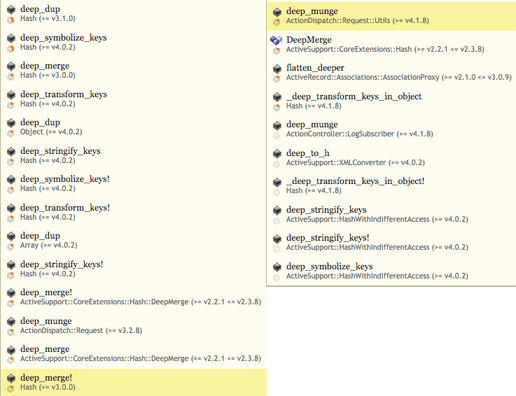
Examples of
deep
tails in links
Strong parameters that permit hashes with unknown keys looking ugly
Here, best syntaxis suggestions were ignored:
params.require(:product).permit(:name).permit!(:data)
params.require(:product).permit(:name, :data => Hash)
Instead, we should use a tap.
1 2 3 4 5 | def permitted_params params.require(:product).permit(:name).tap do |while_listed| while_listed[:data] = params[:product][:data] end end |
Why do people create whitelists of parameters in wrappers?
Ok, I understand that it’s good for security reasons. But if you give up support of your wrapper, you will make people spend time on investigating, forking, fixing, pull requesting, waiting for your reaction, and, as a result, writing in a Gemfile.
gem 'neglected_gem', github: 'me/neglected', :ref => '4aded'
And don’t forget to use :ref
—instead of getting the latest master of a forked repository—to save some trees. 🌲
Do you really need to rename scope variables to synonyms?
The less we change the names of variables with the same value, the less we look at the screen, and the more we save trees. 🌲
1 2 | scope: item: '=ngModel' |
1 2 | scope: obligatory: '=required' |
A lot of duplicating of the same functionality in libraries, especially in JavaScript
When I see articles such as “I’m a web developer, and I’ve been stuck with the simplest app for the last 10 days,” I think it’s a bad signal. It would be better to join efforts and create something amazing instead of the classic.
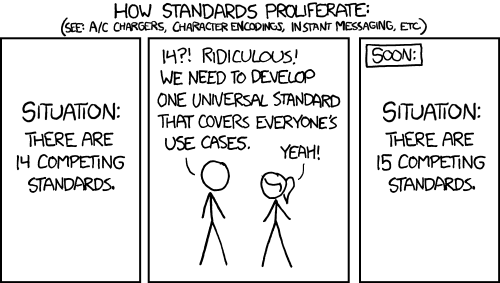
xkcd standards (Image credit)
Supported technologies
I understand that there are a lot of activity: ES2015
, TypeScript
, HTML5
, and CSS4
. In addition, a variety of pre- and postprocessors allow you to use new syntax and new methods. Should we, though, use them if they are not yet supported by any browser, so we can employ supported technologies instead of writing the same functionality? It just makes our machines compile slow, complicates debugging, and forces using function mappings.
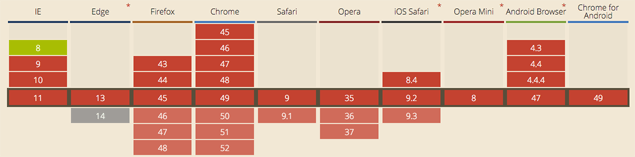
A weak quality of plug-ins
Once, I decided to use a plug-in for the library. I didn’t want to utilize the functionality that relies on multiple dependencies of this plug-in. However, I had to add all the dependencies, because it wasn’t optional. Anyway, this plug-in didn’t work as expected, and I decided to write my own simple plug-in that has just one required dependency. And I realized that the last 10 versions still have serious bugs even though there were several issues on GitHub about it. I could accept some bugs if it were for a young library, but not the one with 700+ commits and a lot of tests.
Another example is complexity
I just wanted to save some time and use a library for building trees in the document object model (DOM) based on the deep
object. In the beginning, it worked fine. When I was asked to add the Select all functionality, I was surprised that I should pass a flatten list to options in my tree to mark all items selected instead of just adding the _selected: true
flag. But it still worked fast and fine until I was asked to expand all nodes. While passing the same flatten list to options for expanding a node, the library did not work properly. Some nodes were expanded, some not. When I click to expand one node, another one collapses! After investigating sources, I couldn’t believe that such simple functionality needs so much code. Dear colleagues, please save some trees 🌲 and write short, simple, and understandable code.